Field of particle positions. More...
#include <field.hpp>
Public Types | |
using | vec = LCS::Vector< T, Dim > |
Public Member Functions | |
Position (unsigned nx, unsigned ny) | |
void | SetAll (const std::vector< T > &xrange, const std::vector< T > &yrange) |
void | SetAll (const T &xmin, const T &xmax, const T &ymin, const T &ymax) |
void | SetAll (Tensor< vec, Dim > &data) |
auto | Get (const unsigned i, const unsigned j) const |
auto & | GetRange (const unsigned axis) |
void | Update (Velocity< T, Dim > &vel, T delta) |
void | InitializeOutOfBoundTensor () |
bool | IsOutOfBound (const unsigned i, const unsigned j) |
void | SetBound (const T &xmin, const T &xmax, const T &ymin, const T &ymax) |
![]() | |
Field (unsigned nx, unsigned ny) | |
auto & | GetAll () const |
auto | GetTime () const |
void | ReadFromFile (const std::string &file_name) |
void | SetAll (Tensor< Vector< T, Size >, Dim > &data) |
void | UpdateTime (const T time) |
void | WriteToFile (const std::string &file_name) const |
Additional Inherited Members | |
![]() | |
const unsigned | nx_ |
const unsigned | ny_ |
Tensor< Vector< T, Size >, Dim > | data_ |
T | time_ |
Detailed Description
template<typename T, unsigned Dim>
class LCS::Position< T, Dim >
Field of particle positions.
This class is used for representing positions (displacements) of particles. It is a subclass of Field.
- Template Parameters
-
T Numeric data type of the field. Dim Dimension of the field (2 or 3).
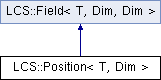
Constructor & Destructor Documentation
|
inline |
Constructor for initializating the position field.
- Parameters
-
nx The number of points in -direction.
ny The number of points in -direction.
Member Function Documentation
|
inline |
Get the positions of a grid point.
- Parameters
-
i Index in -coordinate.
j Index in -coordinate.
- Returns
- Tuple contains
- and
-coordinates of a given grid point.
|
inline |
Get the range of coordinates for a given axis.
- Parameters
-
axis Integer that represents an axis (0 for -axis, 1 for
-axis).
|
inline |
Initialize the Tensor that contains out-of-bound information. All points are assumed to be inside the boundary.
|
inline |
Check if a point is out of the boundary.
- Parameters
-
i Index in -coordinate.
j Index in -coordinate.
- Returns
- A bool that shows if the point is out of boundary.
|
inline |
|
inline |
Set data values of the field using the coordinates of end points. The grid is assumed to be an uniform Cartesian grid when using this function. This is a overloaded function.
- Parameters
-
xmin The minimum -coordinate.
xmax The maximum -coordinate.
ymin The minimum -coordinate.
ymax The maximum -coordinate.
|
inline |
Set data values of the field. This function would class the function with the same name in the base class.
|
inline |
Set the out boundary of the field.
- Parameters
-
xmin The minimum -coordinate of the out boundary.
xmax The maximum -coordinate of the out boundary.
ymin The minimum -coordinate of the out boundary.
ymax The maximum -coordinate of the out boundary.
|
inline |
Update positions of each grid point using a velocity field. OpenMP is used here for accelerating the calculations.
- Parameters
-
vel Velocity field contains the velocity information of each grid point. delta Time step for updating the positions.
The documentation for this class was generated from the following file:
- src/field.hpp