Class for flow fields with discrete data. More...
#include <flow.hpp>
Public Member Functions | |
DiscreteFlowField (unsigned nx, unsigned ny, unsigned data_nx, unsigned data_ny) | |
DiscreteFlowField (unsigned nx, unsigned ny) | |
void | SetVelocityFileNamePrefix (const std::string prefix) |
void | SetVelocityFileNameSuffix (const std::string suffix) |
void | ReadDataVelocityFromFile (Velocity< T, Dim > &data_vel) |
void | SetCurrentVelocity () |
void | SetDirection (const Direction direction) |
void | CopyInitialPositionToCurrentPosition () |
auto & | DataPosition () |
auto & | CurrentDataVelocity () |
void | SetDataDelta (const T delta) |
void | SetDataTimeRange (const T t1, const T t2) |
![]() | |
FlowField (unsigned nx, unsigned ny) | |
auto & | InitialPosition () |
auto & | CurrentPosition () |
virtual Velocity< T, Dim > & | CurrentVelocity () |
auto | GetTime () |
auto | GetDirection () |
void | SetDelta (const T delta) |
void | SetStep (const unsigned step) |
void | SetInitialTime (const T time) |
void | UpdateTime () |
void | UpdateTime (const T time) |
void | Run () |
Additional Inherited Members | |
![]() | |
const unsigned | nx_ |
const unsigned | ny_ |
T | delta_ |
T | initial_time_ |
T | current_time_ |
unsigned | step_ |
Direction | direction_ = Forward |
std::unique_ptr< Position< T, Dim > > | initial_pos_ |
std::unique_ptr< Position< T, Dim > > | current_pos_ |
std::shared_ptr< Velocity< T, Dim > > | current_vel_ |
Detailed Description
template<typename T, unsigned Dim = 2>
class LCS::DiscreteFlowField< T, Dim >
Class for flow fields with discrete data.
This class is used for representing flow fields with discrete data which typically come from experiments or CFD simulations.
- Template Parameters
-
T Numeric data type of all the numeric values in the flow. Dim Dimension of the flow field (2 or 3).
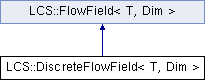
Constructor & Destructor Documentation
|
inline |
Constructor for initializating the discrete flow field.
- Parameters
-
nx The number of grid points in -direction for calculation.
ny The number of grid points in -direction for calculation.
data_nx The number of grid points in -direction in the input flow data.
data_ny The number of grid points in -direction in the input flow data.
|
inline |
Constructor for initializating the discrete flow field when the input data and calculation data have the same resolution.
- Parameters
-
nx The number of grid points in -direction.
ny The number of grid points in -direction.
Member Function Documentation
|
inlinevirtual |
Copy the initial position to the current position.
Reimplemented from LCS::FlowField< T, Dim >.
|
inline |
Get the current velocity field of the input data.
|
inline |
Get the Position field of the input data.
|
inline |
Read velocity data from a file.
- Parameters
-
data_vel Velocity fields that contains the input velocity data from a file.
|
inlinevirtual |
Calculate and set the current velocities of the flow particles from temporal and spatical interpolations of the velocity data.
Reimplemented from LCS::FlowField< T, Dim >.
|
inline |
Set the time difference between two adjacent data files.
- Parameters
-
delta Time difference between two adjacent data files.
|
inline |
Set the time range of the data files.
- Parameters
-
t1 One end point of the data time range. t2 The another end point of the data time range.
|
inlinevirtual |
Set the advection direction.
- Parameters
-
direction Direction for particle advection.
Reimplemented from LCS::FlowField< T, Dim >.
|
inline |
Set the prefix of the file names of the input velocity data.
- Parameters
-
prefix A string contains the prefix of file names.
|
inline |
Set the suffix of the file names of the input velocity data.
- Parameters
-
suffix A string contains the prefix of file names.
The documentation for this class was generated from the following file:
- src/flow.hpp